Making A Player Character is one of the most Important things to do in ANY game development process. Here is how to do it in both 3D and 2D
There are a couple steps we will do for this character.
Step One: Node Tree
To Start, we need to make the correct setup for the character. the node tree goes like this:
- CharacterBody3d
- MeshInstance3d
- CollisionShape3d
- Node3d (call this node “Head”)
- Camera3d
For the mesh instance 3d, set the shape to the weird pill shape thing
For the Collision Shape 3d, select that shape as well.
ok. now its time to map the controls
To start, click on the label called project at the top of godot
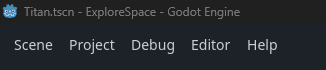
click on project settings, then on input map

Finally, set it up like this.
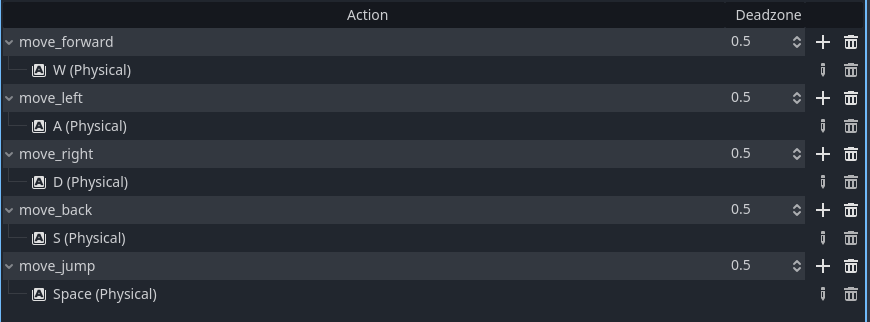
Now, time for the code.
extends CharacterBody3D
const SPEED = 200
const JUMP_VELOCITY = 20
const SENSITIVITY = .001
#Get the gravity from the project settings to be synced with RigidBody nodes.
var gravity = ProjectSettings.get_setting("physics/3d/default_gravity")
@onready var head = $Head
@onready var camera = $Head/Camera3D
func _ready():
Input.set_mouse_mode(Input.MOUSE_MODE_CAPTURED)
func _unhandled_input(event):
if event is InputEventMouseMotion:
head.rotate_y(-event.relative.x * SENSITIVITY)
camera.rotate_x(-event.relative.y * SENSITIVITY)
camera.rotation.x = clamp(camera.rotation.x, deg_to_rad(-40), deg_to_rad(60))
func _physics_process(delta):
# Add the gravity.
if not is_on_floor():
velocity.y -= gravity * delta
# Handle jump.
if Input.is_action_just_pressed("move_jump"):
velocity.y = JUMP_VELOCITY
# Get the input direction and handle the movement/deceleration.
# As good practice, you should replace UI actions with custom gameplay actions.
var input_dir = Input.get_vector("move_left", "move_right", "move_back", "move_forward")
#Calculate the forward and right directions based on the camera orientation.
var forward_dir = -camera.global_transform.basis.z
var right_dir = camera.global_transform.basis.x
#Calculate the direction the player should move.
var direction = (forward_dir * input_dir.y + right_dir * input_dir.x).normalized()
if direction:
velocity.x = direction.x * SPEED
velocity.z = direction.z * SPEED
else:
velocity.x = move_toward(velocity.x, 0, SPEED)
velocity.z = move_toward(velocity.z, 0, SPEED)
move_and_slide()
Attach this as a script to the character body 3d node. I will now break the code down for you.
First, to modify any player attributes, modify the constants at the top
const SPEED = 200
const JUMP_VELOCITY = 20
const SENSITIVITY = .001
Speed = Player Speed
Jump_Velocity = how high the player jumps
Sensitivity = Mouse Sensitivity
thats actually all you need to know to use this nvm sorry lol
Leave a Reply